intro
Let’s learn the foundations of SQL query optimization, its significance, and its application. Get better at writing queries that supercharge your database performance!
SQL query optimization involves improving the efficiency of SQL queries to ensure faster execution and reduced resource consumption.
Well-optimized queries can significantly improve database performance, reduce server load, and provide quicker results to end users. All demonstrations are going to be made with DbVisualizer—the database client with the highest user satisfaction in the world.
SQL Query Optimization: What Is It?
SQL query optimization is the process of improving the speed and efficiency of SQL queries. The goal of query optimization in SQL is to locate and fix performance issues, which typically involves altering the database schema, creating indexes, or adjusting the query.
The ultimate objective is to minimize the time and resources needed to run a query. As a result, applications that depend on that query will operate more efficiently.
Why the Need for SQL Query Optimization?
In database management, optimizing queries is of incredible importance . Why is this so? Well, there are many reasons but the sole purpose of optimizing SQL queries is to make applications run faster and smoother by cutting down the execution time of your queries and at the end, offering a better user experience.
Let’s dig into some of these reasons!
Performance Impact
Unoptimized queries can take seconds or even minutes to execute, while optimized versions might complete in milliseconds. This directly affects user experience, especially in customer-facing applications.
For instance, when a customer is waiting for their order history to load, the difference between a 3-second and 300-millisecond response time can determine whether they stay on your site.
Resource Utilization
Think of an unoptimized query like a car engine running at full throttle to maintain highway speed–it gets the job done but wastes fuel and strains the engine. A well-optimized query is like a properly tuned engine that delivers the same performance with minimal resource consumption.
Cost Implications
Another need for query optimization is cost implications, especially with cloud services. Every unnecessary CPU cycle or I/O (Input/Output) operation adds to your bill. Cloud costs can be significantly reduced by simply optimizing your most frequently run queries.
Scalability
Scalability is critical in the life of a database developer because what works today might fail tomorrow as your data grows. A query that's fast enough with 10,000 records could bring your system to a halt when you reach a million records. Optimization is not just about current performance–it's about future-proofing your application.
Best Practices and Techniques for SQL Query Optimization
Optimize your SQL queries with some proven practices and techniques for better performance and efficiency .
1. Use Indexes on the Frequently Queried Columns
With proper indexing, you can drastically reduce your data retrieval time. Create indexes on frequently queried columns that are used in GROUP BY
, ORDER BY
, WHERE
clauses, and JOIN
conditions. Note also, to avoid over-indexing as it increases write operation costs.
Example of an indexed column:
1
CREATE INDEX idx_customer_name ON customers(name);
For more details, read our guide on 10x query performance with a database index.
2. Avoid Using the DISTINCT Keyword
SQL DISTINCT
is a clause used to remove duplicate rows from a result set, ensuring only unique values are returned. It is particularly useful when retrieving distinct values from a column with many duplicates.
However, using DISTINCT
on large datasets may introduce performance overhead, as the database must process and eliminate duplicates. Proper indexing and query optimization can help mitigate this. Instead of relying on DISTINCT
to filter duplicates, it's often more efficient to structure queries to return unique data from the start, using indexing, constraints, or optimized joins.
While DISTINCT
is a valuable tool, it can be resource-intensive. When possible, optimizing queries to prevent duplicates at the source is a smarter approach.
Consider an example query that uses DISTINCT
:
1
SELECT DISTINCT first_name, last_name
2
FROM customers
3
WHERE orders.status = 'Completed';
The statement above selects all unique first and last name combinations of customers who have completed their orders.
Rewriting an equivalent query without DISTINCT
:
1
SELECT first_name, last_name
2
FROM customers
3
WHERE orders.status = 'Completed'
4
GROUP BY first_name, last_name;
These two queries will get identical results, however, when no aggregation is performed, DISTINCT
is generally more efficient than GROUP BY
because GROUP BY
is optimized for grouping and aggregation rather than simple deduplication. In cases where we need to count orders per customer (e.g., COUNT(o.order_id)
), GROUP BY
becomes the preferred choice.
3. Use Temporary Tables
Another way to SQL query optimization tip is to use temporary tables. A temporary table, also known as temp table, is a kind of table that enables a database to store and handle intermediate results. In a database management system, it is used in a particular session within the context of a specific session.
When used in queries, temporary tables are helpful for storing and modifying interim results. Because if less data needs to be processed, performance can be enhanced.
Also, instead of writing a single large query with multiple joins and subqueries, breaking it into smaller steps using temporary tables can improve readability and performance.
This is the example of a query without a temp table:
1
SELECT c.name, COUNT(o.order_id) AS order_count
2
FROM customers c
3
JOIN orders o ON c.customer_id = o.customer_id
4
WHERE o.status = 'Completed'
5
GROUP BY c.name
6
HAVING COUNT(o.order_id) > 5;
In the query above, there is the repeated scan of orders
, which potentially slows down execution.
Using a temp table, you can get:
1
CREATE TEMPORARY TABLE temp_completed_orders AS
2
SELECT customer_id, COUNT(order_id) AS order_count
3
FROM orders
4
WHERE status = 'Completed'
5
GROUP BY customer_id;
6
7
SELECT c.name, t.order_count
8
FROM customers c
9
JOIN temp_completed_orders t ON c.customer_id = t.customer_id
10
WHERE t.order_count > 5;
Here,:
4. Avoid SELECT
It is always advisable to select the exact number of columns you need. Selecting unnecessary columns with the *
operator increases the amount of data transferred and processed, and hence makes the operation less efficient.
Consider this example:
1
SELECT * FROM customers
Instead of doing the above, rather do:
1
SELECT first_name, last_name FROM customers
How EXPLAIN Works in SQL
The SQL EXPLAIN
command displays a query's execution plan. Specifically, EXPLAIN
gives details on how the query optimizer will carry out the query, which is key for SQL query optimization. The information returned by the command includes:
Keep in mind that this information varies depending on how EXPLAIN
is implemented by the DBMS.
In most databases, the EXPLAIN
command can be executed as follows:
1
EXPLAIN
2
<SQL_QUERY>
EXPLAIN SQL Example for SQL Query Optimization
Consider the EXPLAIN
query below:
1
EXPLAIN
2
SELECT name, managerId
3
FROM Teams
4
WHERE ballondorCandidateId IS NOT NULL
5
GROUP BY name, managerId
Before executing the query, create a sample table, index and populate it with data with the query below in DbVisualizer:
1
-- Step 1: Create the table 'teams'
2
CREATE TABLE teams (
3
id SERIAL PRIMARY KEY,
4
name TEXT NOT NULL,
5
managerId INT NOT NULL,
6
ballonDorCandidateId INT -- This can be NULL
7
);
8
9
-- Step 2: Indexing for performance
10
CREATE INDEX idx_teams_managerId ON teams (managerId);
11
CREATE INDEX idx_teams_ballonDorCandidateId ON teams (ballonDorCandidateId) WHERE ballonDorCandidateId IS NOT NULL;
12
CREATE INDEX idx_teams_name_managerId ON teams (name, managerId);
13
14
-- Step 3: Insert sample data
15
INSERT INTO teams (name, managerId, ballonDorCandidateId) VALUES
16
('team A', 701, 1),
17
('team B', 702, 2),
18
('team C', 701, NULL),
19
('team A', 701, 3),
20
('team D', 703, NULL),
21
('team B', 702, 4),
22
('team E', 704, 5);
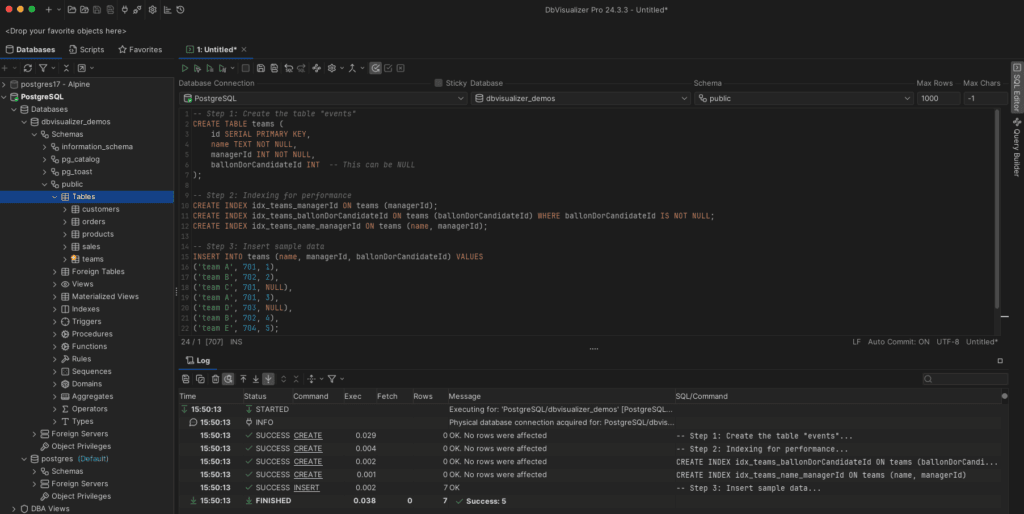
Now, after executing the query, we should see this:
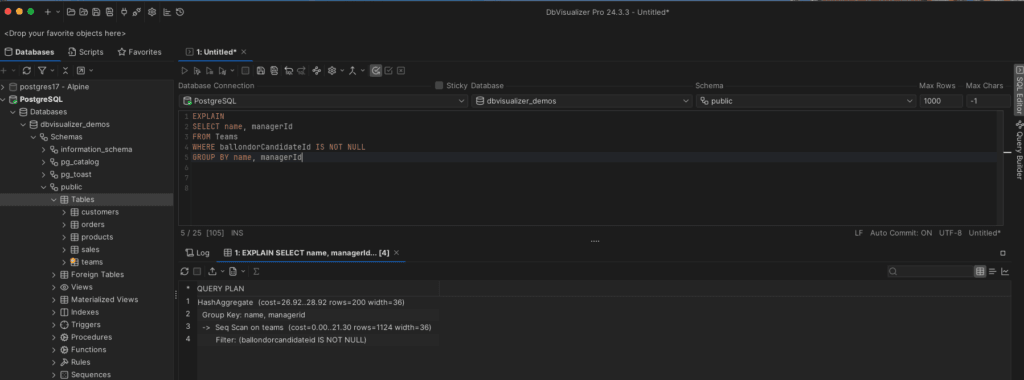
Note that the query is not executed by EXPLAIN
. Because of that, you must use EXPLAIN ANALYZE
if you want to obtain information on the query execution time.
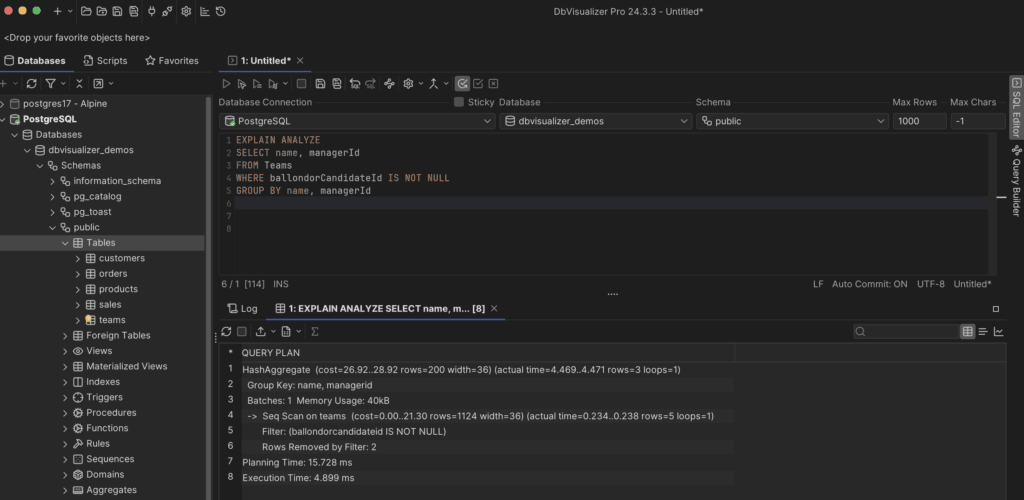
As you can see, EXPLAIN ANALYZE
additionally provides valuable information on the amount of time needed for preparation and query execution.
The Explain Plan Feature in DbVisualizer
You can visually examine how a query is handled by the database with DbVisualizer's Explain Plan feature. The Explain Plan runs your query and logs the execution strategy the database came up with.
Find out if the database is using the right indexes and joining your tables in the most efficient way by simply looking at the plan. This enables you to comprehend the background operations of your DBMS and optimize your queries appropriately.
To analyze a query with the Explain Plan in DbVisualizer:
Step 1: Write out the query in DbVisualizer.
Step 2: Click on the “Execute Explain Plan” button in the toolbar shown below
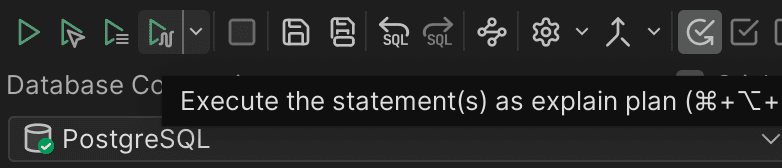
Step 3: Finally, examine the query output.
In the example that follows, we are going to learn to use DbVisualizer’s Explain Plan. Time to dive into it.
DbVisualizer’s Explain Plan Example
Assume you want to run the query in a PostgreSQL database with the Explain plan:
1
SELECT * FROM Teams T
2
WHERE T.managerId IS NOT NULL
3
ORDER BY managerId ASC
This query does not follow any SQL query optimization best practices and techniques presented earlier. Let’s run it in the Explain Plan.
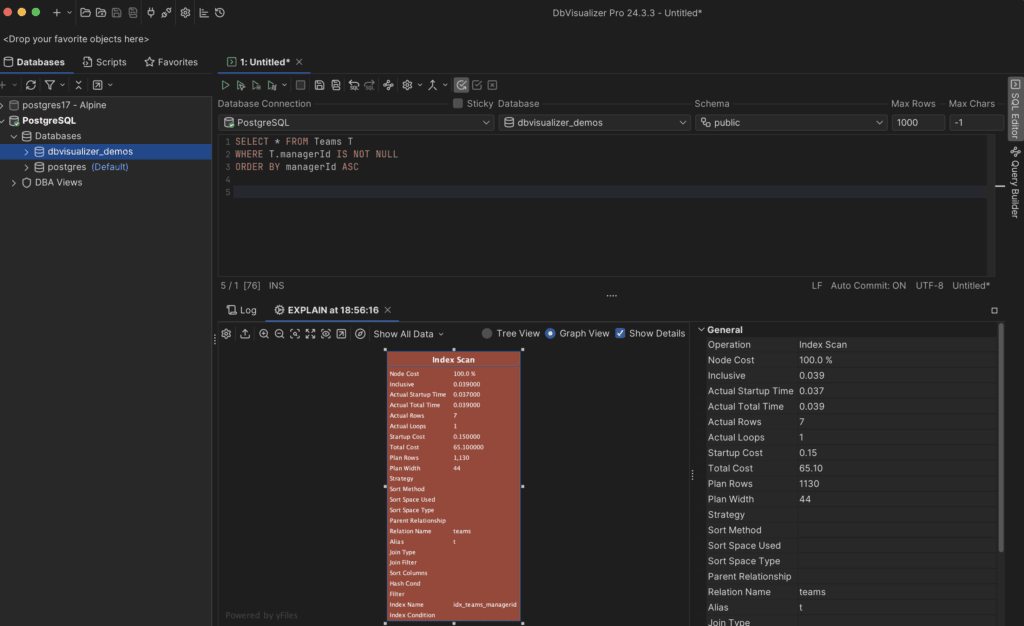
You can see the execution plan as a chart in the “Graph View” section in DbVisualizer. It gives you a visual representation of how your query will be executed. While in “Tree View,” the information is in a much condensed form. Note that the Total Cost
is 65.0
Total Cost
tells you the overall computational cost required to execute a query. This involves the amount of memory used, the number of CPU cycles required, and/or the number of disk I/O operations performed. The value for the total cost is inversely proportional to the efficiency of a query (i.e., the lower the total cost value is, the more efficient a query is).
Next, you can implement the practices shared earlier in this blog to make your query more efficient. This include:
And that is all for this blog!
Conclusion
SQL query optimization is an important measure to make queries faster. In this blog, you learned some useful measures for SQL performance optimization. In detail, you also learned what the EXPLAIN
command is and how it can help you analyze an execution plan.
Despite its strength, the command can be challenging to use and comprehend. Fortunately for you, the Explain Plan feature is included in DbVisualizer! This enables you to see visually the actions the DBMS takes to process the query, the indexes it uses, and the areas where performance might be enhanced.
Remember that we are offering a free 21-day trial for DbVisualizer, so grab the deal, share this article with your circle, and until next time!
FAQs
What is SQL query optimization?
SQL query optimization is the process of improving the performance of database queries by minimizing execution time and resource usage. This involves techniques like indexing, reducing joins, using proper filtering conditions, and avoiding unnecessary computations.
How do indexes improve query performance?
Indexes speed up data retrieval by allowing the database engine to locate rows quickly, rather than scanning the entire table. Proper indexing reduces query execution time, especially for large datasets.
When should I use temporary tables for query optimization?
Use temporary tables when you need to store intermediate results, reduce redundant calculations, or break down complex queries into smaller, more manageable steps. This can improve performance by limiting the number of records processed in joins and aggregations.
How can I speed up a slow-running SQL query?
First, analyze the query execution plan to identify your bottlenecks. Learn to avoid using SELECT *
, consider using WHERE
clauses to filter rows early, and uses indexes the right way, etc. Those are just a few of the things to look at!